Introduction
In web development, interactivity is a key aspect of creating engaging and user-friendly web applications. JavaScript, the programming language of the web, provides powerful tools to enable interactivity, and one of the fundamental mechanisms for achieving this is through event listeners. In this article, we’ll explore event listeners in JavaScript, their role in web development, and how to use them to respond to user actions.
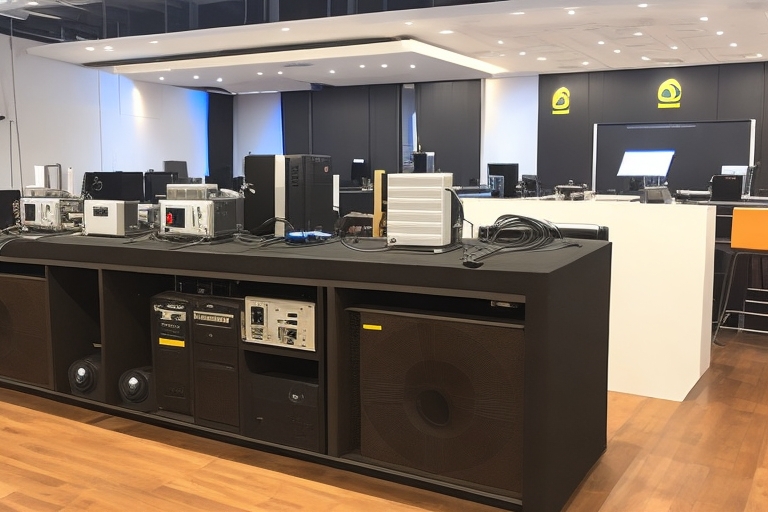
What Are Event Listeners?
Event listeners are JavaScript functions that wait for specific events to occur on HTML elements and then respond to those events. Events can be user interactions, such as clicks, mouse movements, and keyboard inputs, or they can be generated by the browser or external sources, such as data loading or timer expirations.
Event listeners are crucial for making web applications interactive because they enable developers to define how the application should react when certain actions or events take place.
Adding Event Listeners to Elements
To add an event listener to an HTML element, you typically follow these steps:
- Select the HTML element: Use JavaScript to select the HTML element to which you want to attach the event listener. This is often done using methods like
getElementById
,querySelector
, orgetElementsByTagName
. - Define the event listener function: Create a JavaScript function that will be executed when the specified event occurs. This function can contain any code you want to run in response to the event.
- Attach the event listener: Use the
addEventListener
method to attach the event listener function to the selected HTML element. You specify both the type of event (e.g., “click,” “mouseover,” “keydown”) and the function to be executed.
Here’s a simple example of adding a click event listener to a button element:
const myButton = document.getElementById("myButton");
function handleClick() {
alert("Button clicked!");
}
myButton.addEventListener("click", handleClick);
Common Event Types
JavaScript supports a wide range of event types that you can listen for, including:
- Mouse events:
click
,mouseover
,mouseout
,mousemove
, and more. - Keyboard events:
keydown
,keyup
,keypress
. - Form events:
submit
,change
,input
. - Focus events:
focus
,blur
,focusin
,focusout
. - Document and window events:
DOMContentLoaded
,load
,resize
,scroll
.
Event Object
When an event occurs, an event object is automatically created and passed to the event listener function. This object contains information about the event, such as the type of event, the target element, and any additional data related to the event.
You can access properties of the event object within your event listener function to gather information about the event and make decisions based on it.
function handleMouseOver(event) {
console.log(`Mouse entered element: ${event.target.tagName}`);
}
Removing Event Listeners
In some cases, you may want to remove an event listener from an element, such as when you no longer need to respond to a specific event. To remove an event listener, you use the removeEventListener
method, specifying the same event type and function that you used when adding the listener.
myButton.removeEventListener("click", handleClick);
Conclusion
Event listeners are a fundamental part of building interactive web applications with JavaScript. They allow you to respond to a wide range of user actions and events, making your web pages dynamic and engaging. By understanding how to add, remove, and work with event listeners, you gain the ability to create web experiences that respond to user input and provide a richer and more interactive user interface. Event listeners are a core tool in the web developer’s toolkit for building modern, user-centric web applications.