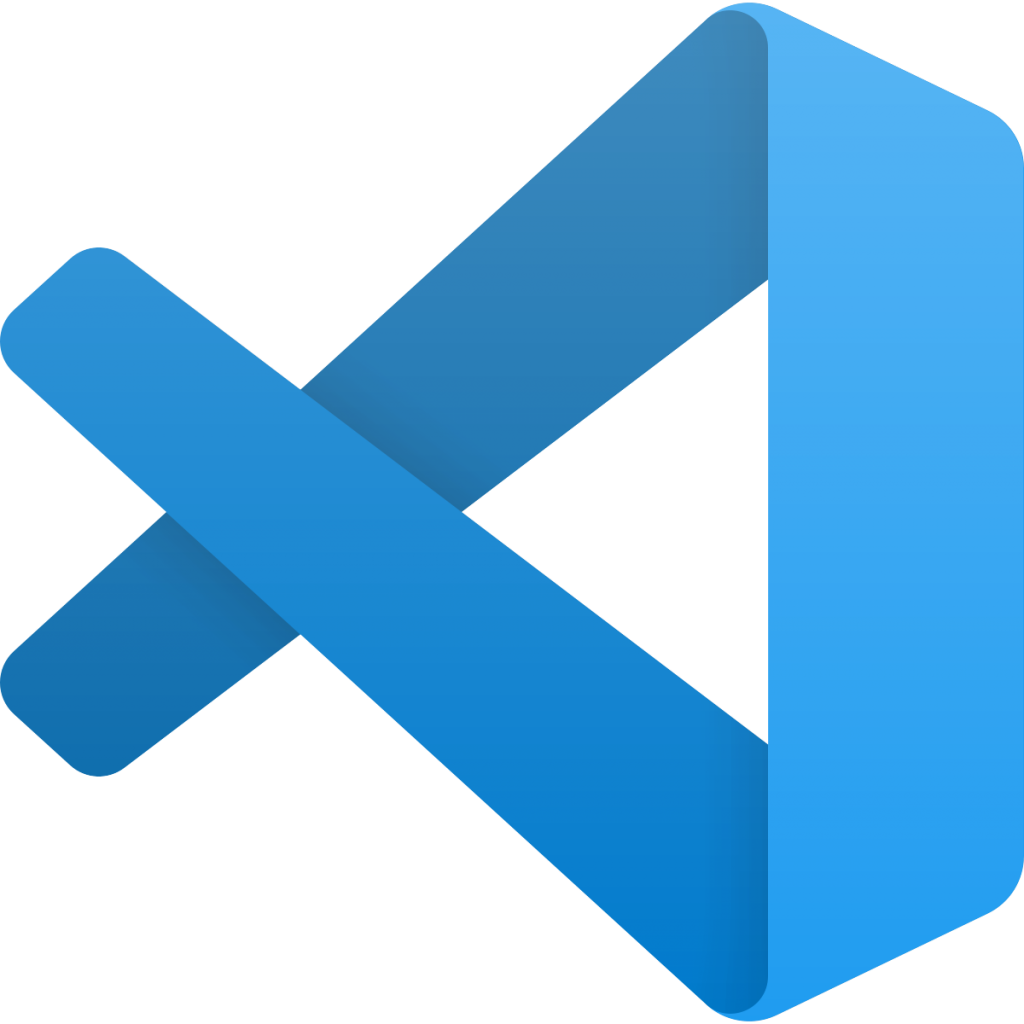
Step1.Install VSCode
If you haven’t already, download and install Visual Studio Code from the official website.
Step2.Install Java Development Kit (JDK)
Make sure you have the Java Development Kit installed on your system. You can download it from the official Oracle website or use OpenJDK. Ensure that you set up the JAVA_HOME
environment variable to point to your JDK installation directory.
Step3.Install Visual Studio Code Extensions
- Open VSCode and go to the Extensions view by clicking on the square icon in the sidebar or by pressing
Ctrl+Shift+X
. - Search for “Extension Pack for Java” and install it. This extension pack includes essential tools for Java development in VSCode.
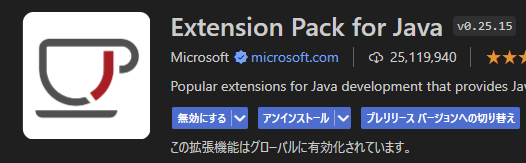
Step4.Install Maven
- Download and install Apache Maven from the official website.
- Follow the installation instructions for your operating system.
- Open a terminal or command prompt.
- Type
mvn -v
and press Enter. - You should see the Maven version and other information if it’s installed correctly.

Step5.Create a Maven Project
- Open VSCode and create a new folder for your Maven project.
- Open a terminal in VSCode by clicking on
Terminal
>New Termina
l. - In the terminal, navigate to your project folder.
- Run the command:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-project -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
- This command generates a basic Maven project structure with a sample Java class and a
pom.xml
file.
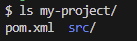
Step6.Add JUnit Dependency to pom.xml
Add the JUnit dependency inside the <dependencies>
section:
(version 3.8.1 may be installed already)
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version> <!-- or any other version -->
<scope>test</scope>
</dependency>
Step7.Install Dependencies
$ mvn install
Step8.Create JUnit Test
Probably AppTest.java was created already. You can add test here.
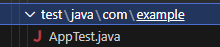
Step9.Run JUnit Tests
Right click the project and execute test.
Or you can execute “mvn test” from console.
$ mvn test